Vue3 介绍
与其它大型框架不同的是,Vue 被设计为可以自底向上逐层应用。Vue 的核心库只关注视图层,不仅易于上手,还便于与第三方库或既有项目整合。
另一方面,当与现代化的工具链以及各种支持类库结合使用时,Vue 也完全能够为复杂的单页应用提供驱动。
声明式渲染
Vue.js 的核心是一个允许采用简洁的模板语法来声明式地将数据渲染进 DOM 的系统:
1 2 3
| <div id="counter"> Counter: {{ counter }} </div>
|
1 2 3 4 5 6 7 8 9
| const Counter = { data() { return { counter: 0 } } }
Vue.createApp(Counter).mount('#counter')
|
除了文本插值,我们还可以像这样绑定元素的 attribute:
1 2 3 4 5
| <div id="bind-attribute"> <span v-bind:title="message"> 鼠标悬停几秒钟查看此处动态绑定的提示信息! </span> </div>
|
1 2 3 4 5 6 7 8 9
| const AttributeBinding = { data() { return { message: 'You loaded this page on ' + new Date().toLocaleString() } } }
Vue.createApp(AttributeBinding).mount('#bind-attribute')
|
处理用户输入
可以用 v-on
指令添加一个事件监听器,通过它调用在实例中定义的方法:
1 2 3 4
| <div id="event-handling"> <p>{{ message }}</p> <button v-on:click="reverseMessage">反转 Message</button> </div>
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| const EventHandling = { data() { return { message: 'Hello Vue.js!' } }, methods: { reverseMessage() { this.message = this.message .split('') .reverse() .join('') } } }
Vue.createApp(EventHandling).mount('#event-handling')
|
所有的 DOM 操作都由 Vue 来处理,你编写的代码只需要关注逻辑层面即可。
Vue 还提供了 v-model
指令,它能轻松实现表单输入和应用状态之间的双向绑定。
1 2 3 4
| <div id="two-way-binding"> <p>{{ message }}</p> <input v-model="message" /> </div>
|
1 2 3 4 5 6 7 8 9
| const TwoWayBinding = { data() { return { message: 'Hello Vue!' } } }
Vue.createApp(TwoWayBinding).mount('#two-way-binding')
|
条件与循环
控制切换一个元素是否显示
1 2 3
| <div id="conditional-rendering"> <span v-if="seen">现在你看到我了</span> </div>
|
1 2 3 4 5 6 7 8 9
| const ConditionalRendering = { data() { return { seen: true } } }
Vue.createApp(ConditionalRendering).mount('#conditional-rendering')
|
v-for
指令可以绑定数组的数据来渲染一个项目列表:
1 2 3 4 5 6 7
| <div id="list-rendering"> <ol> <li v-for="todo in todos"> {{ todo.text }} </li> </ol> </div>
|
1 2 3 4 5 6 7 8 9 10 11 12 13
| const ListRendering = { data() { return { todos: [ { text: 'Learn JavaScript' }, { text: 'Learn Vue' }, { text: 'Build something awesome' } ] } } }
Vue.createApp(ListRendering).mount('#list-rendering')
|
组件化应用构建
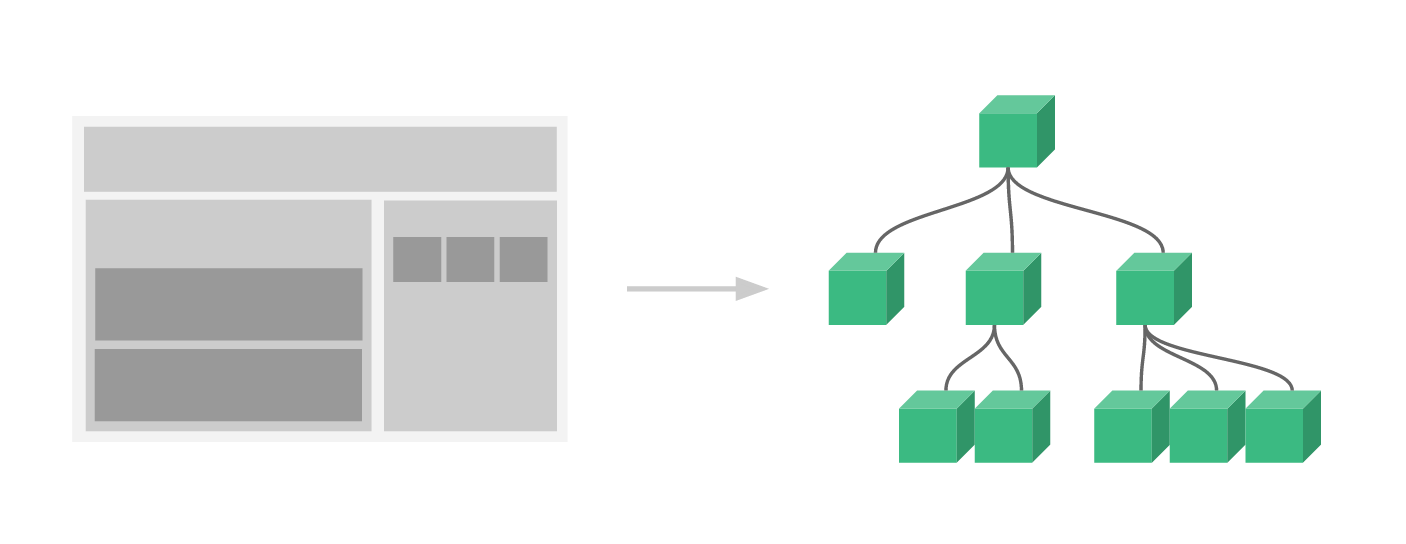
在 Vue 中,组件本质上是一个具有预定义选项的实例。在 Vue 中注册组件很简单:如对 app
对象所做的那样创建一个组件对象,并将其定义在父级组件的 components
选项中:
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| const TodoItem = { template: `<li>This is a todo</li>` }
const app = Vue.createApp({ components: { TodoItem }, ... })
app.mount(...)
|
1 2 3 4
| <ol> <todo-item></todo-item> </ol>
|
我们应该能将数据从父组件传入子组件才对。让我们来修改一下组件的定义,使之能够接受一个 prop:
1 2 3 4
| const TodoItem = { props: ['todo'], template: `<li>{{ todo.text }}</li>` }
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| <div id="todo-list-app"> <ol>
<todo-item v-for="item in groceryList" v-bind:todo="item" v-bind:key="item.id" ></todo-item> </ol> </div>
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
| const TodoItem = { props: ['todo'], template: `<li>{{ todo.text }}</li>` }
const TodoList = { data() { return { groceryList: [ { id: 0, text: 'Vegetables' }, { id: 1, text: 'Cheese' }, { id: 2, text: 'Whatever else humans are supposed to eat' } ] } }, components: { TodoItem } }
const app = Vue.createApp(TodoList)
app.mount('#todo-list-app')
|